Adding a copyright notice to an Apollo GraphQL API
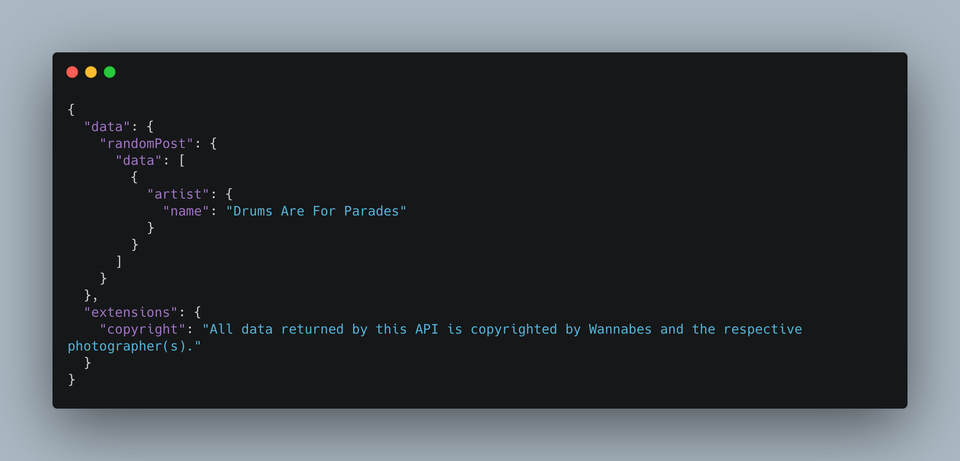
You’re building an open API, but you want to make sure your copyright notice is displayed alongside the data?
While building the backend for our rock photography website, I faced the same problem. I want hobbyists and tinkerers to be able to play with our API, but I want to clarify the copyright terms. We don’t want people stealing our images because our API is open.
Unfortunately, Apollo GraphQL doesn’t offer an easy way to enforce fields to be sent to users.
(bad) Solution 1: add it as a field to the schema
You could add a copyright field to all your types and return it. But since you can’t force clients to fetch this field, this is rather useless.
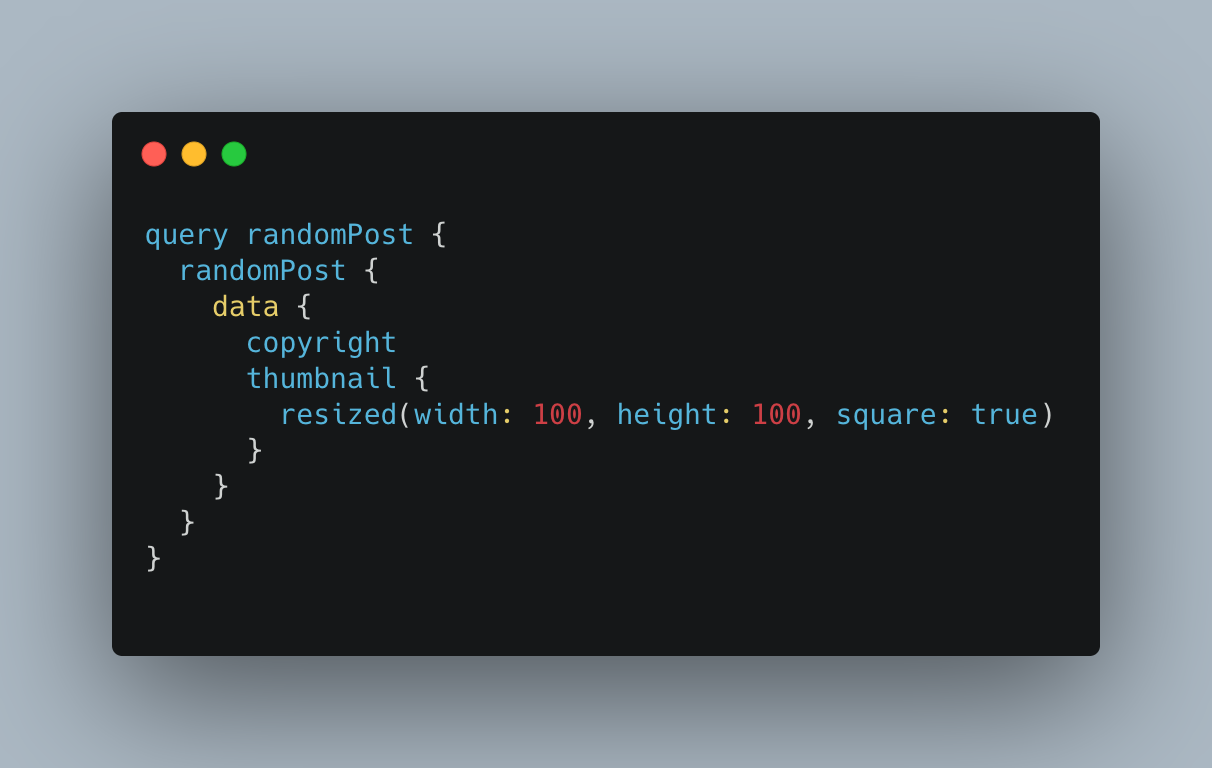
(bad) Solution 2: add it to the headers
You could write middleware to add an X-COPYRIGHT header to all your responses. But you can’t expect clients to inspect every header actively.
(good) Solution 3: return it as data inside the extension object
GraphQL responses can return meta-information inside an object named extensions. Apollo-server uses this object to return tracing info. By writing a simple apollo-server plugin, you can enforce the field:
const CopyrightPlugin = { requestDidStart(requestContext) { return { willSendResponse(context) { context.response.extensions = { ...context.response.extensions, copyright: "All data returned by this API is copyrighted by Wannabes and the respective photographer(s).", }; }, }; },}; const server = new ApolloServer({ ...createSchema(db), debug: false, engine: { reportSchema: true, }, cors: true, playground: true, introspection: true, tracing: true, plugins: [ CopyrightPlugin] });
You could theoretically return it as an extra field on all your responses (skipping the extensions object), but it feels cleaner returning it as part of the extensions object.
That’s it. You can use this technique to do other cool things, like returning rate limits, uptime, or other nifty stats.
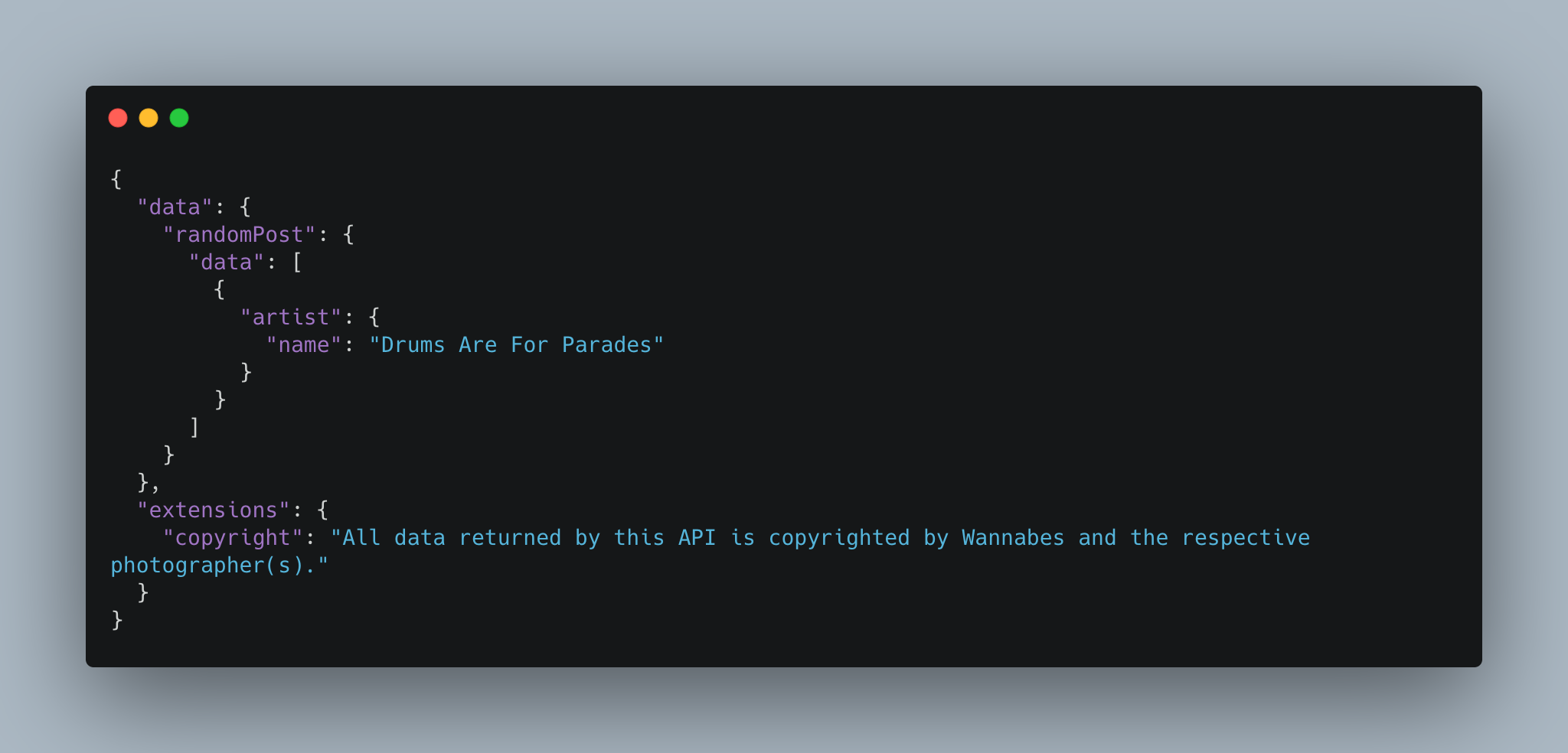
Member discussion